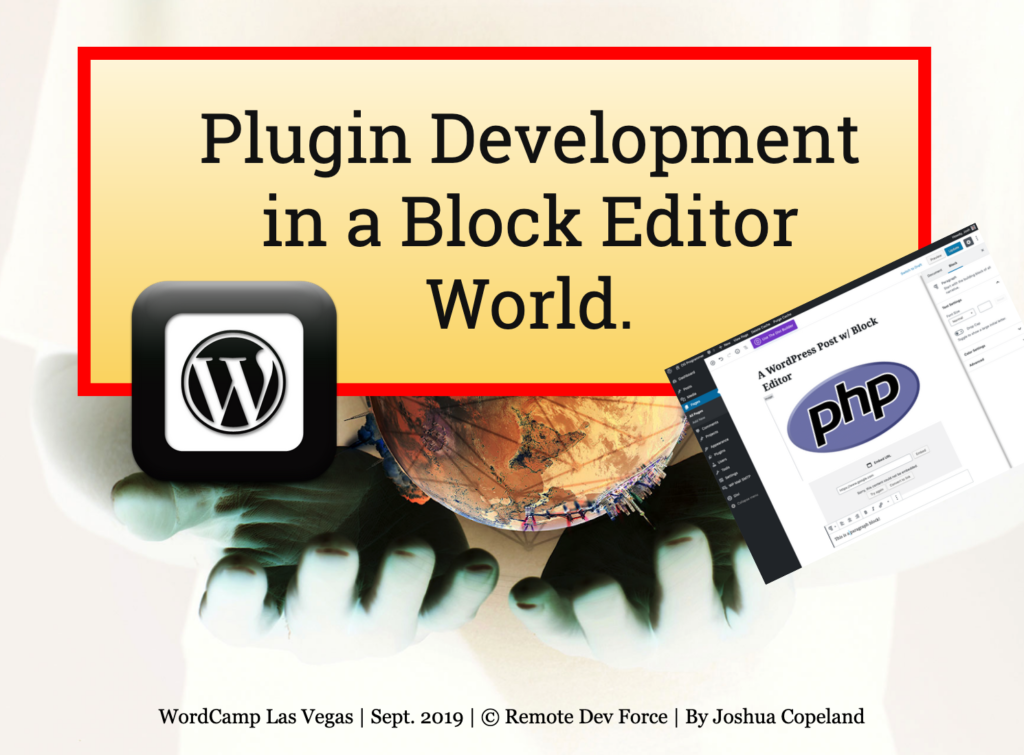
In this article, we will cover how to create a custom Gutenberg Block in WordPress. We created some examples on our GitHub:
https://github.com/RemoteDevForce/wordpress-block-type-example
Whether you like it or not, WordPress page design and content creation now relies on Gutenberg by default. The new WordPress Block Editor has caused some confusion and inadvertently some push back. We now live in a “Block Editor World” so we must learn what a block is and how they work. The days of one giant WYSIWYG editor for a post are over. The new compartmentalized nature of Blocks are the cornerstone to building WordPress sites the right way. Using React, it keeps blocks decoupled from your other blocks/components.
In the examples, there is a Stock Ticker Block example we created that simply accesses the price from Yahoo! public finance data. First you need to create a plugin directory for your block to live in. In the plugin, you’ll want to create a plugin.php
and a block.js
file. In the plugin PHP file, put the following code.
<?php
function loadMyBlock()
{
wp_enqueue_script(
'rdf-custom-dynamic-blocks-example',
plugin_dir_url(__FILE__) . 'block.js',
array('wp-blocks', 'wp-i18n', 'wp-editor'),
true
);
}
add_action('enqueue_block_editor_assets', 'loadMyBlock');
function stockPriceOutput($props)
{
// Get the price
$response = wp_remote_get('https://query1.finance.yahoo.com/v8/finance/chart/' . trim($props['symbol']));
if (empty($response['body'])) {
error_log('An error occurred when trying to fetch stock prices.');
return 'ERROR';
} else {
$data = json_decode($response['body'], true);
if (!empty($data['chart']['result'][0]['meta']['regularMarketPrice'])) {
$stockPrice = $data['chart']['result'][0]['meta']['regularMarketPrice'];
}
return '<h3 style="color: #000;">' . $props['symbol'] . ' : $' . $stockPrice . '</h3>';
}
}
register_block_type('rdf/stock-block', array(
'render_callback' => 'stockPriceOutput',
));
// Metadata
function myStockBlockMeta()
{
register_meta('post', 'symbol', array('show_in_rest' => true, 'type' => 'string', 'single' => true));
}
add_action('init', 'myStockBlockMeta');
Once you have that all tucked into the plugin.php file, add the following to the block.js
file.
// STOCK PRICE BLOCK
wp.blocks.registerBlockType('rdf/stock-block', {
title: 'Stock Block',
icon: 'chart-area',
category: 'common',
attributes: {
symbol: {type: 'string'},
},
edit: function(props) {
function updateContent(event) {
props.setAttributes({symbol: event.target.value})
}
return wp.element.createElement(
"div",
null,
wp.element.createElement(
"h3",
null,
"My Stock Block"
),
wp.element.createElement("input", { type: "text", value: props.attributes.symbol, onChange: updateContent })
);
},
save: function(props) {
// This will make a callback to PHP when you return null
return null;
}
});
Activate your plugin and your custom block will now be listed. Go create a post and look for a new block called “Stock Block” and put a stock ticker into the box. Save the post and view the page and you will see the stock ticket and the price!
Creating blocks is super easy once you get the hang of wiring everything up with WP & React. There are a few ways to make this block more resilient:
- Cache API calls so remote web calls don’t execute every page load.
- Return JSON instead of HTML from PHP.
- Better CSS Styles (Green for gains and Red for losses)
- Add a chart or other data from the API.
If you want to skip and just run the example, go checkout the example Readme for details on running the docker setup.
Click here for the Google Slides
Here are other resources that can help when learning block development:
Video
Tools
Documentation
Tutorial
If you need any custom blocks created, contact us at Remote Dev Force to get it built.
Recent Comments